OpDisplayFilter
Overview
The OpDisplayFilter performs a user-specified operation
on two input buffers: input1
and input2
.
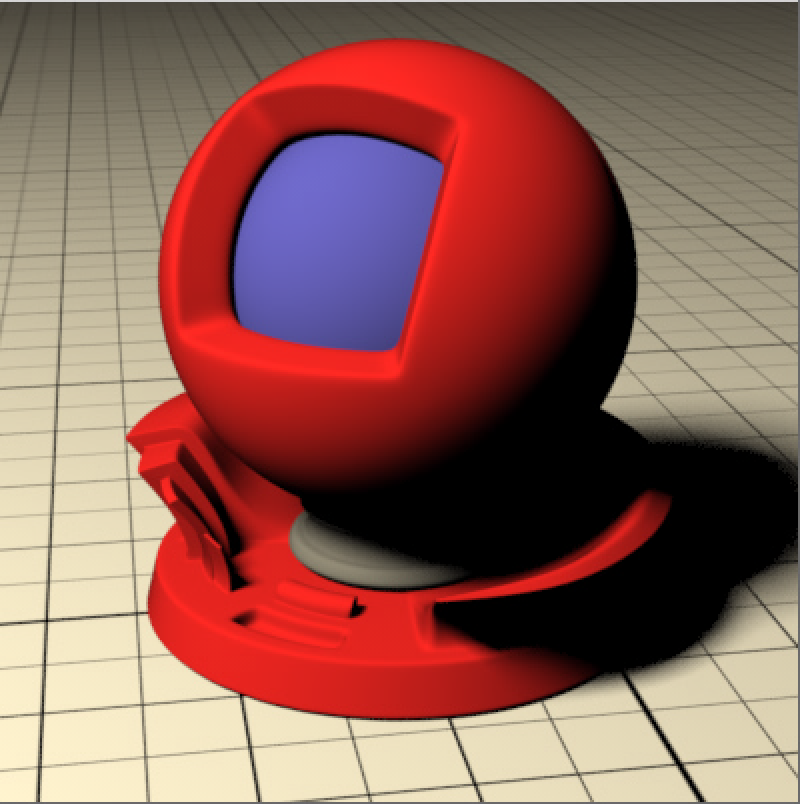
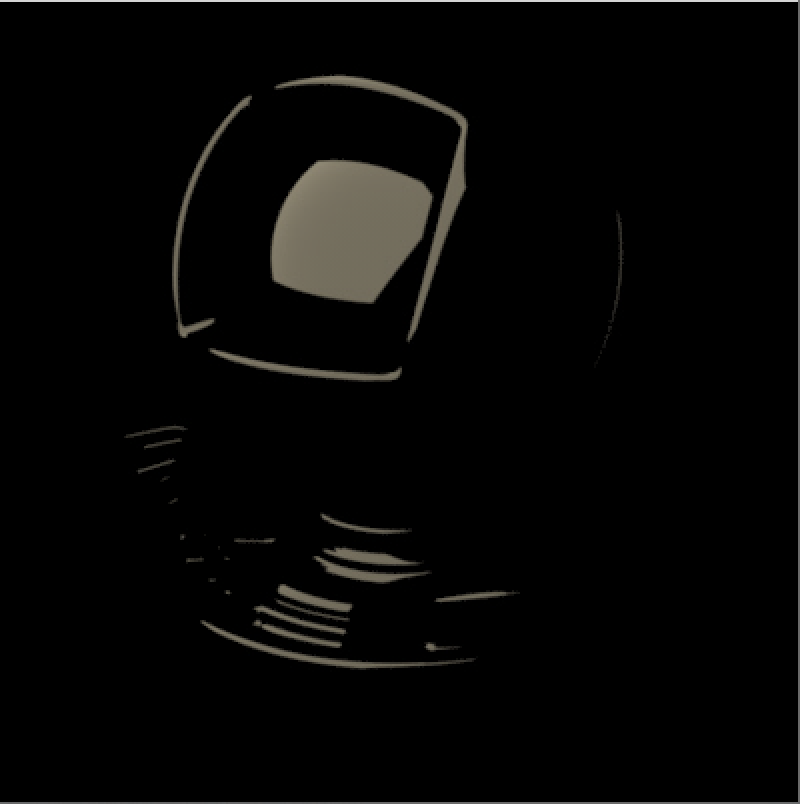
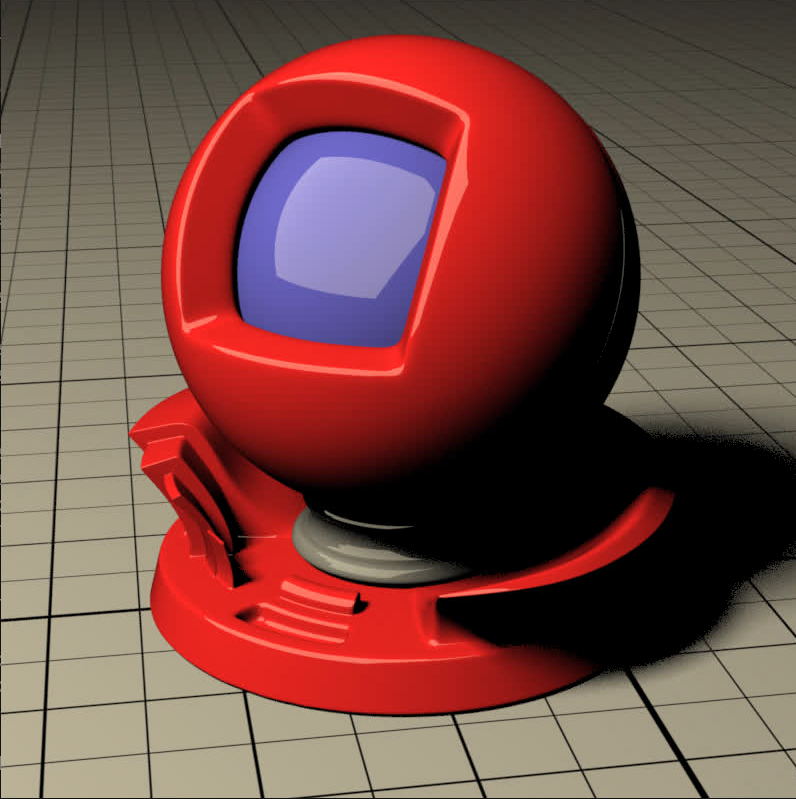
Attribute Reference
Advanced attributes
invert_mask
Bool
default: False
Invert the value of the mask
mix
Float
default: 1.0
Blend [0,1] between input and output
General attributes
input1
RenderOutput
default: None
First RenderOutput; required
input2
RenderOutput
default: None
Second RenderOutput; optional
mask
RenderOutput
default: None
RenderOutput used to mask the output, revealing input1
operation
Int enum
0 = “add” (default)
1 = “subtract”
2 = “multiply”
3 = “divide”
4 = “min”
5 = “max”
6 = “power”
7 = “cross”
8 = “dot”
9 = “modulo”
10 = “greater_than”
11 = “greater_than_or_equal”
12 = “less_than”
13 = “less_than_or_equal”
14 = “equal”
15 = “not_equal”
16 = “and”
17 = “or”
18 = “xor”
19 = “invert”
20 = “normalize”
21 = “abs”
22 = “ceil”
23 = “floor”
24 = “length”
25 = “sine”
26 = “cosine”
27 = “round”
28 = “acos”
29 = “not”
Operation to use on the input(s)
Examples
local glossy = RenderOutput("/output/glossy") {
["file_name"] = "result_tmp.exr",
["result"] = "light aov",
["light_aov"] = "glossy"
}
local diffuse = RenderOutput("/output/diffuse") {
["file_name"] = "result_tmp.exr",
["result"] = "light aov",
["light_aov"] = "diffuse"
}
local add = OpDisplayFilter("/display/add") {
["input1"] = diffuse,
["input2"] = glossy,
["operation"] = "add",
}
RenderOutput("/output/add") {
["file_name"] = "result0.exr",
["result"] = "display filter",
["display_filter"] = add,
["channel_name"] = "add"
}