NoiseMap_v2
Overview
NoiseMap creates procedural noise based on Ken Perlin’s Improved Noise (2002), a lattice gradient noise. It can create noise using a classic square grid or a simplex grid.
Flow Noise
When using the simplex grid, this node also implements Perlin’s flow noise, creating an appearance of advection that’s perceptually different from scrolling 4D noise.
Order of Operations:
When changing settings of this map, they’re applied in this order:
- Noise Calculation
- Bias
- Gain
- Smoothstep
- Amplitude
- Invert
Attribute Reference
4D attributes
time
Float bindable
default: 0.0
If use 4D noise is on, this is the value for the 4th dimension
use_4D_noise
Bool
default: False
If on, 4-dimensional noise is used instead of 3-dimensional
Adjustment attributes
bias
Float bindable
default: 0.5
Bias the noise towards 0 or 1
gain
Float bindable
default: 0.5
Apply gain to the noise
invert
Bool
default: False
Invert the final pattern
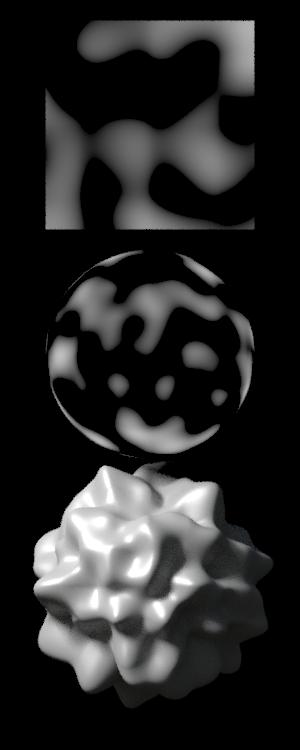
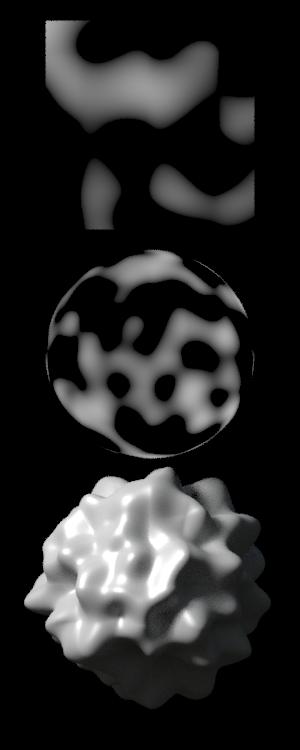
smoothstep
Vec2f bindable
default: [ 0, 1 ]
min/max values between which the smoothstep will interpolate
use_smoothstep
Bool
default: False
Put the noise value through a smoothstep function defined by min/max
Flow Noise attributes
flow_advection_rate
Float bindable
default: 0.0
Rate of advection for flow noise
flow_angle
Float bindable
default: 0.0
Angle of rotation for flow noise
Space attributes
camera
Camera
default: None
camera used to define camera and screen space
input_texture_coordinates
Vec3f bindable
default: [ 0, 0, 0 ]
User specified UVs
object_space
Geometry
default: None
Directly connect object to use that object's space
space
Int enum
0 = “render”
1 = “camera”
2 = “world”
3 = “screen”
4 = “object” (default)
5 = “reference”
6 = “texture”
7 = “input texture coordinates”
8 = “hair_surface_uv”
9 = “hair_closest_surface_uv”
The space to calculate the noise in
Transform attributes
rotation
Vec3f bindable
default: [ 0, 0, 0 ]
Rotates the noise in space based on the specified rotation order
rotation_order
Int enum
0 = “xyz” (default)
1 = “xzy”
2 = “yxz”
3 = “yzx”
4 = “zxy”
5 = “zyx”
Order in which to apply the euler rotations
scale
Vec3f bindable
default: [ 1, 1, 1 ]
Vector to scale the noise non-proportionally
transformation_order
Int enum
0 = “srt”
1 = “str”
2 = “rst”
3 = “rts”
4 = “tsr” (default)
5 = “trs”
Order in which to apply the translation, rotation, and frequency
translation
Vec3f bindable
default: [ 0, 0, 0 ]
Translation of the noise in space
General attributes
amplitude
Float bindable
default: 1.0
Intensity of the noise
color
Bool
default: False
Outputs RGB noise
color_A
Rgb bindable
default: [ 0, 0, 0 ]
The color value at 0 noise
color_B
Rgb bindable
default: [ 1, 1, 1 ]
The color value at 1 noise
distortion
Float bindable
default: 0.0
Warp input coordinate space with single noise level before looking up noise
distortion_noise_type
Int enum
0 = “perlin classic” (default)
1 = “perlin simplex”
Type of noise to use for distortion.
frequency_multiplier
Float bindable
default: 1.0
Scalar multiplier for the frequency vector
lacunarity
Float bindable
default: 2.0
Multiplier on the noise frequency per level
max_level
Float bindable
default: 1.0
Number of octaves of noise to add together for the final result
noise_type
Int enum
0 = “perlin classic” (default)
1 = “perlin simplex”
Type of noise to use. Simplex grid activates Flow Noise Angle and Advection
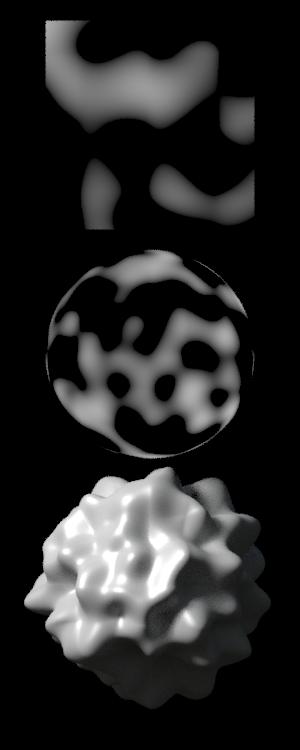
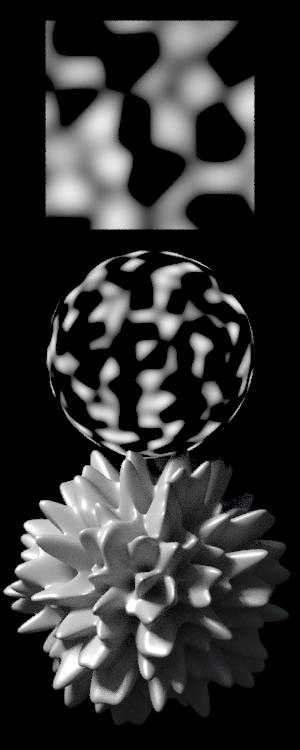
persistence
Float bindable
default: 0.5
Multiplier on the noise amplitude per level
seed
Int
default: 0
The seed for the random number generator