ProjectCameraMap_v2
Overview
ProjectCameraMap_v2 uses a camera frustum to directly apply a texture to a material. This is useful for techniques such as decals and background mattes.
ProjectCameraMap, by comparison, creates texture coordinates that are used as inputs to other maps.
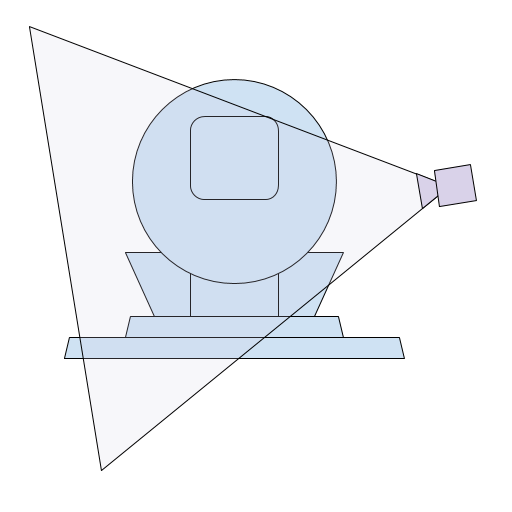
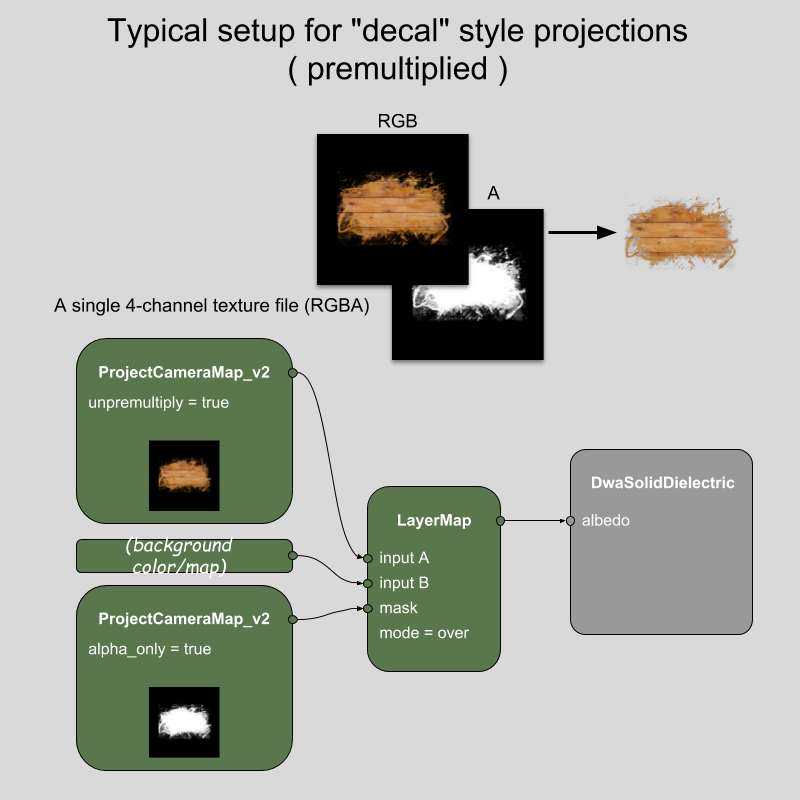
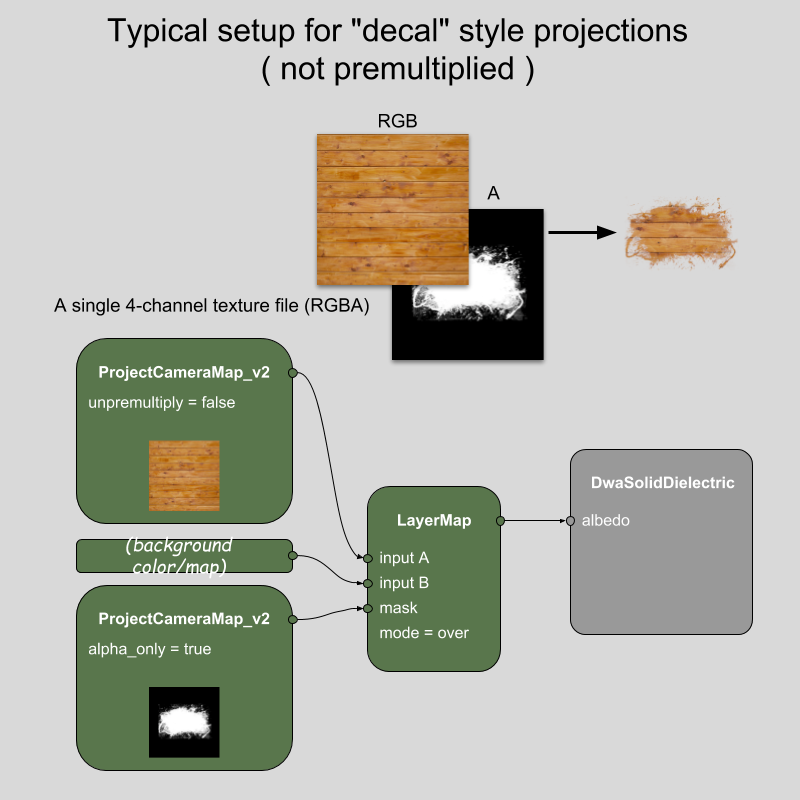
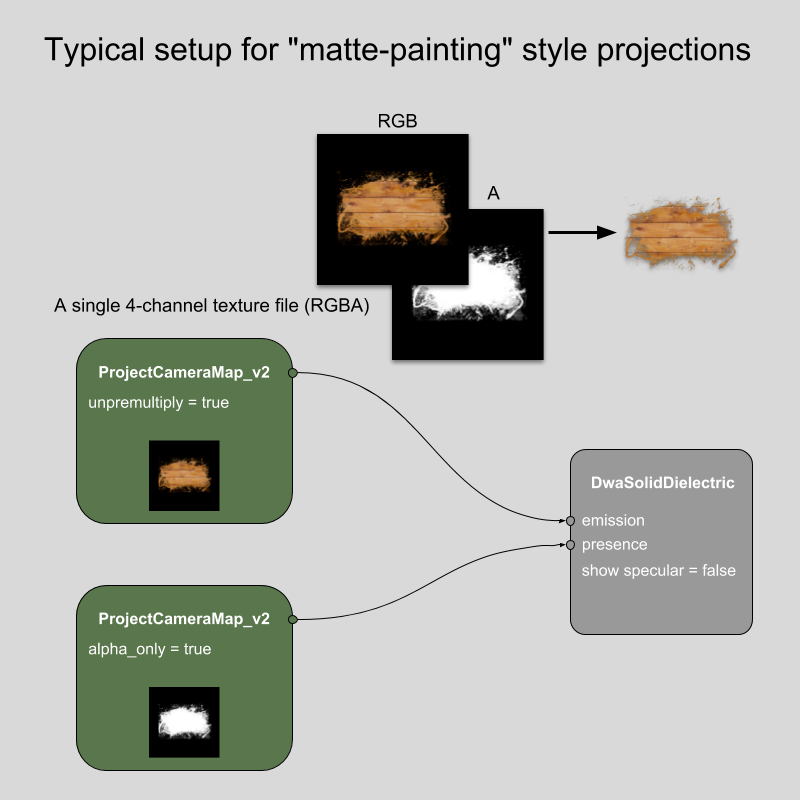
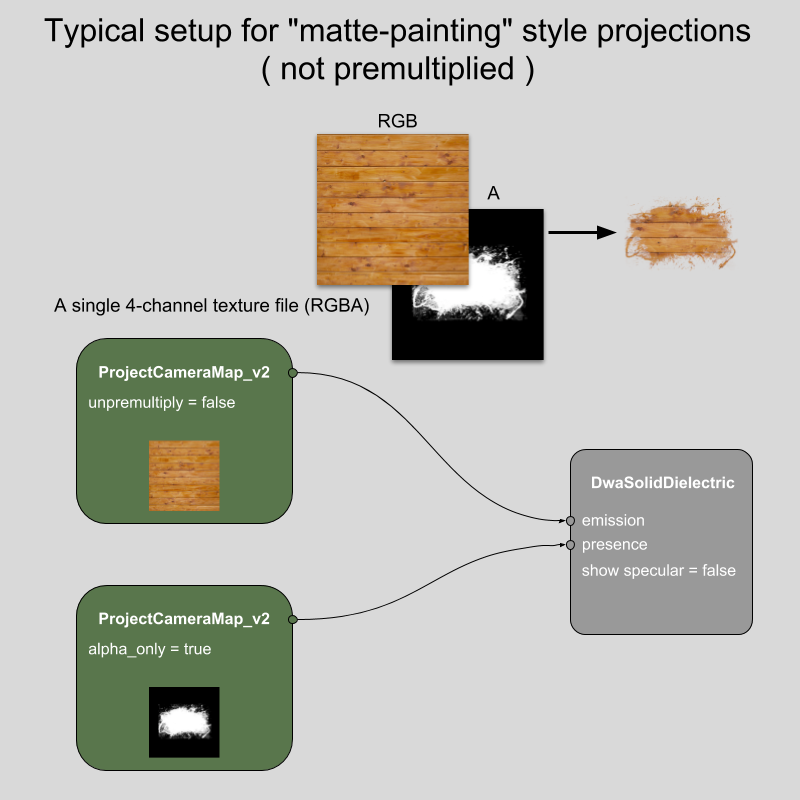
Attribute Reference
General attributes
alpha_only
Bool
default: False
When enabled, the alpha channel is returned instead of RGB
aspect_ratio_source
Int enum
0 = “from texture” (default)
1 = “custom”
Whether to use the image and pixel aspect ratio of the texture being projected, or a custom aspect ratio
black_outside_projection
Bool
default: True
Toggles whether projections appear outside the 0-1 uv range of the projector
custom_aspect_ratio
Float
default: 1.0
Custom aspect ratio for the projected texture
gamma
Int enum
0 = “off”
1 = “on”
2 = “auto” (default)
Controls application of gamma to images (off -0, on - 1, auto - 2). Auto will apply gamma decoding to 8-bit images
project_on_back_faces
Bool
default: False
Toggles whether camera projections appear on back faces.
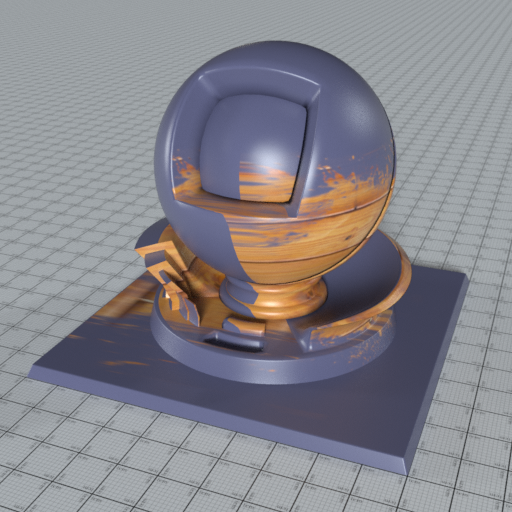
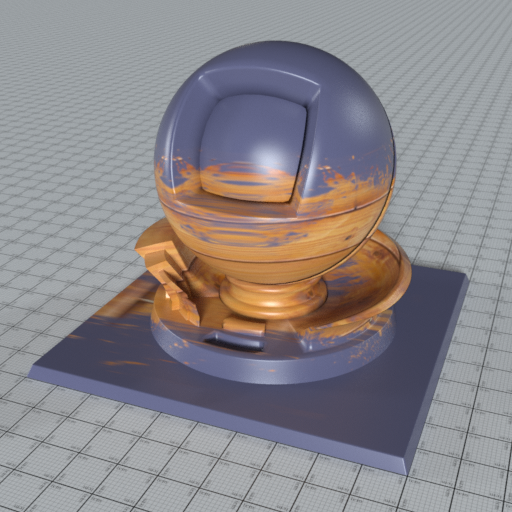
projector
Camera
default: None
The camera to project from
texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
unpremultiply
Bool
default: False
When enabled, the rgb channels are divided by the alpha channel (where non-zero)
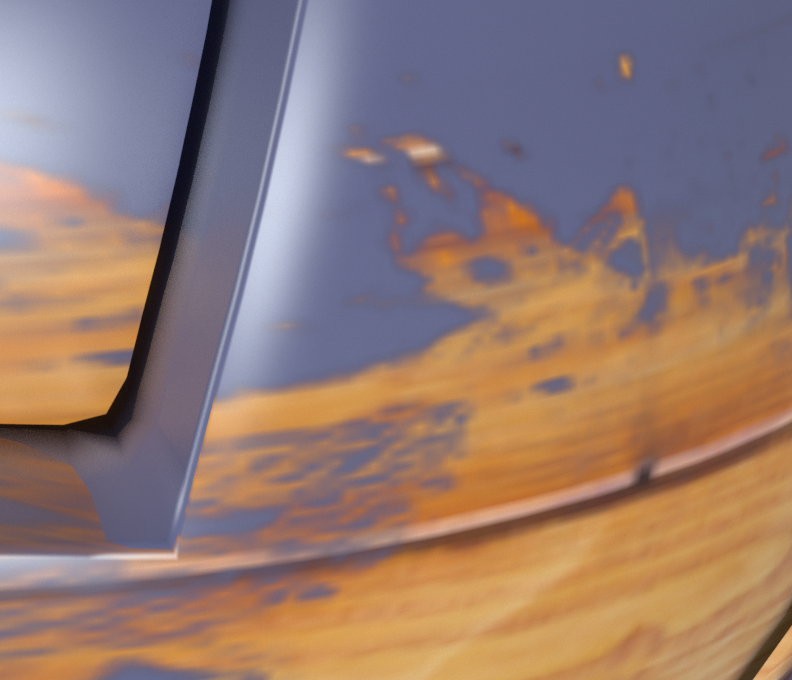
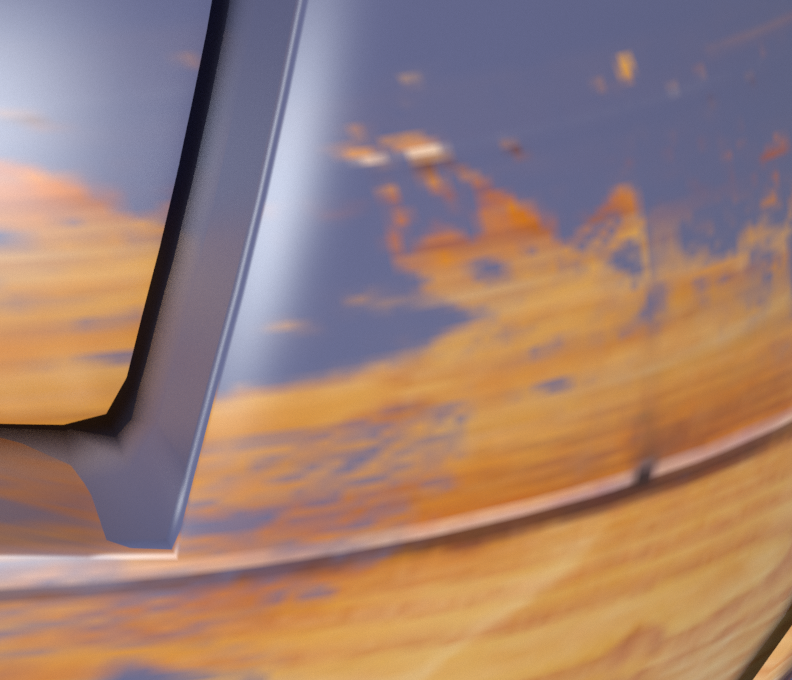
use_reference_space
Bool
default: False
Use reference space
Examples
local projCam1 = Camera("projCam1")
{
["node xform"] = translate(0, 3, 75) * rotate(-5, 1, 0, 0) * rotate(90, 0, 1, 0),
["focal"] = 150,
["film width aperture"] = 24,
}
local projMap = ProjectCameraMap_v2("projMap") {
["projector"] = projCam1,
["texture"] = "myTexture.tx",
["project_on_back_faces"] = false,
}
local mtl1 = DwaSolidDielectricMaterial("mtl1") {
["show diffuse"] = false,
["show specular"] = false,
["show emission"] = true,
["emission"] = bind(projMap, Rgb(1,1,1)),
}