BarnDoorLightFilter
Overview
The BarnDoorLightFilter functions like a barn door in stage lighting. Typically there are four flaps attached to a light that shape the lighting by restricting where the light can shine.
![]() |
---|
Example of a Barn Door (in black) attached to a stage light. |
If the flaps were stitched to each other, the ends of the flaps would form a rectangular portal that constrains the light.
This light filter operates by simulating such a portal, called the flap opening, shown in gray below:
The flap opening can be moved, resized, rotated, rounded, colored, and blurred, with varying blur per side. Here is a rough overview of the various shaping parameters.
The edge expands outwards and there are controls to scale the size of each edge.
![]() |
---|
edge size and per-edge scaling animation |
There are two modes of the Barn Door, analytical and physical.
- In physical mode, the ray between the shading point and the light is checked to see if it passes through the Barn Door rectangular portal. Light rays masked by the portal are darkened.
- In analytic mode, the calculation is the same but the end of the ray on the light is replaced by the singular position of the Barn Door (a single point). It treats the light as a point light for filter shadowing.
![]() ![]() |
![]() ![]() |
![]() |
---|---|---|
analytical mode | physical mode | no filter |
There are two projection types, perspective and orthographic. These are mainly useful for analytical mode, shown below.
![]() ![]() |
![]() ![]() |
![]() |
---|---|---|
perspective projection | orthographic projection | no filter |
The modes affect the shape of the light beam. In physical mode, the projection type determines how big the flap opening is:
- In the perspective projection type, the flap opening size scales with
projector_focal_distance
(roughly maintaining the same solid angle / cone size). - In the orthogonal projection type, the flap opening remains a fixed size. Apart from this, the projection type does not matter.
Attribute Reference
Properties attributes
color
Rgb
default: [ 1, 1, 1 ]
Color within the Barn Door lit region. For each color channel 0 is full shadow and 1 is no shadow.
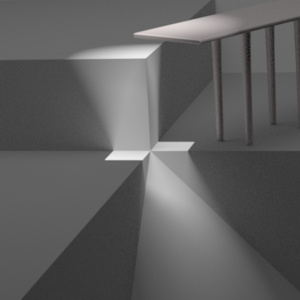
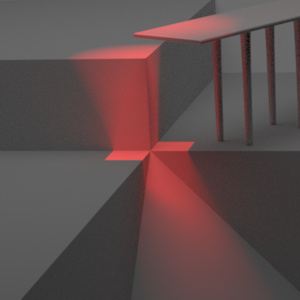
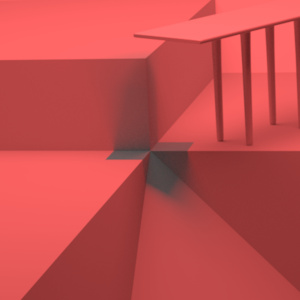
invert = true
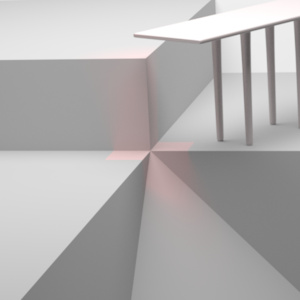
density = 0.5
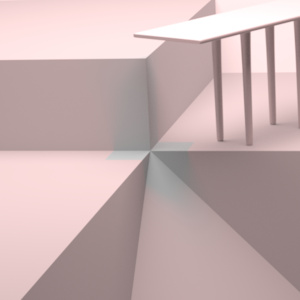
invert = true
density = 0.5
density
Float
default: 1.0
Fades the filter effect. 0 means no effect (like having no filter), and 1 means full effect.
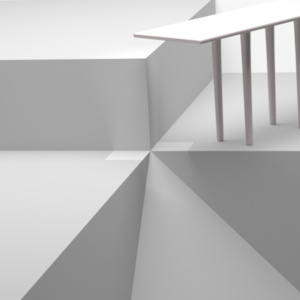
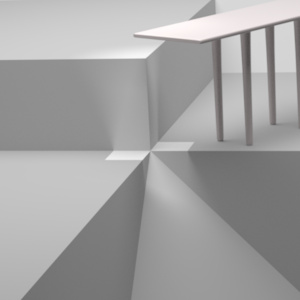
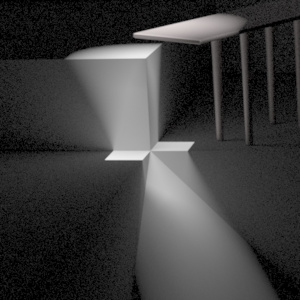
edge
Float
default: 0.0
The size of the transition zone from the rounded box to the outside, as a proportion of width (or height, whichever is smaller).
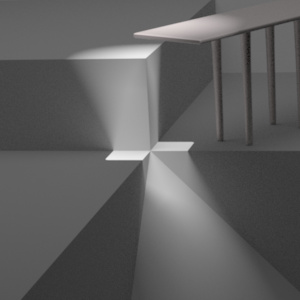
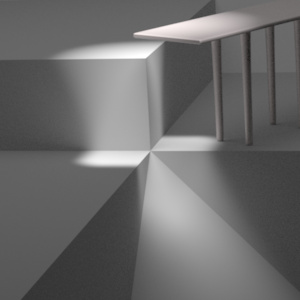
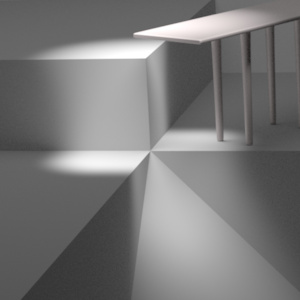
edge_scale_bottom
Float
default: 1.0
The scale factor for the bottom edge.
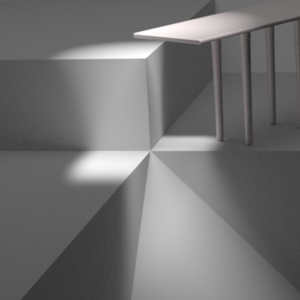
edge_scale_left
Float
default: 1.0
The scale factor for the left edge.
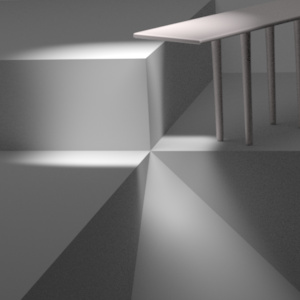
edge_scale_right
Float
default: 1.0
The scale factor for the right edge.
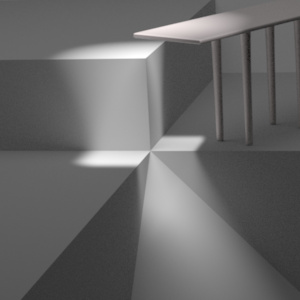
edge_scale_top
Float
default: 1.0
The scale factor for the top edge.
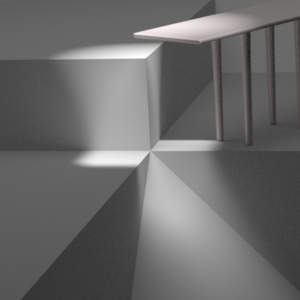
invert
Bool
default: False
Swap application of the filter from inside the Barn Door to outside.
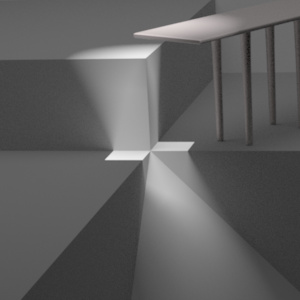
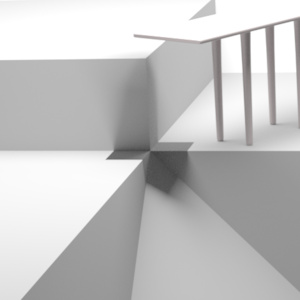
mode
Int enum
0 = “analytical” (default)
1 = “physical”
Analytical mode allows light to shading points that project to the flap opening. Physical mode allows light whose direction goes through the flap opening.
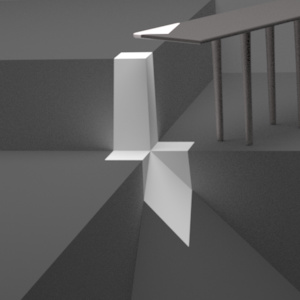
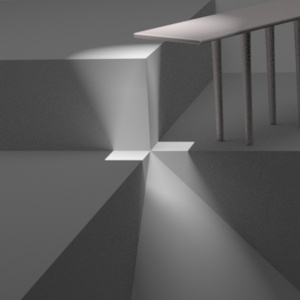
node_xform
Mat4d blurrable
default: [ [ 1, 0, 0, 0 ], [ 0, 1, 0, 0 ], [ 0, 0, 1, 0 ], [ 0, 0, 0, 1 ] ]
The transform of the filter.
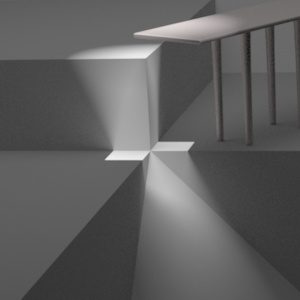
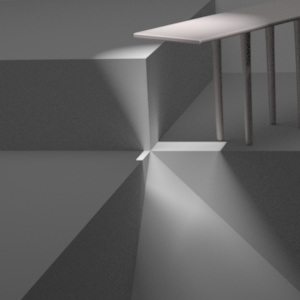
node_xform = rotate(-90,1,0,0) * translate(-2,9,-6)
pre_barn_distance
Float
default: 0.5
The distance from the BarnDoorLightFilter that the pre_barn_mode control takes effect.
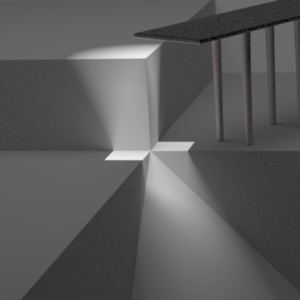
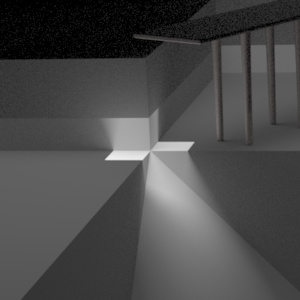
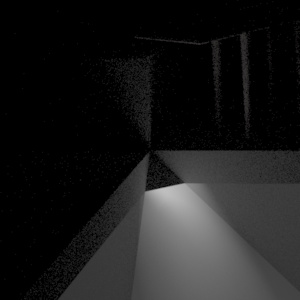
pre_barn_mode
Int enum
0 = “black”
1 = “white”
2 = “default” (default)
Force the region before the pre_barn_distance to be fully filtered (black), not filtered at all (white), or treated the same as elsewhere (default).
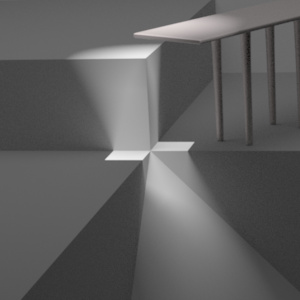
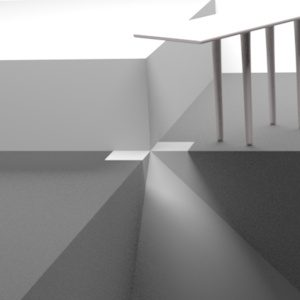
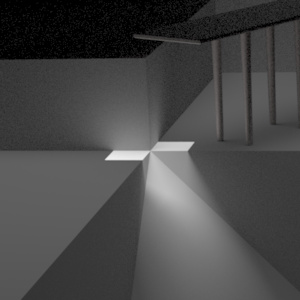
See Also
projector_focal_distance
Float
default: 30.0
The distance of the flap opening from the projector origin. Ignored for orthographic projection.
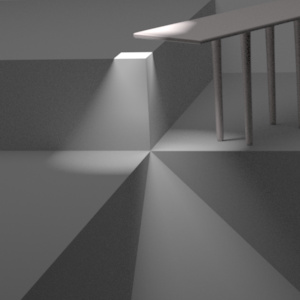
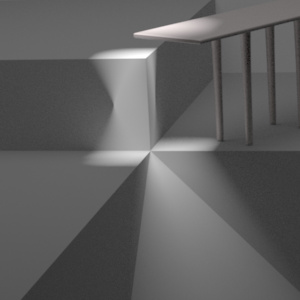
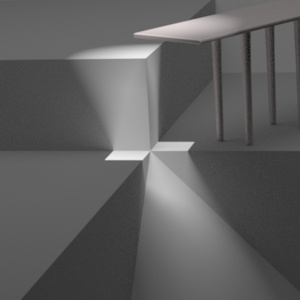
projector_height
Float
default: 1.0
The height of the frustum at distance 1.0.
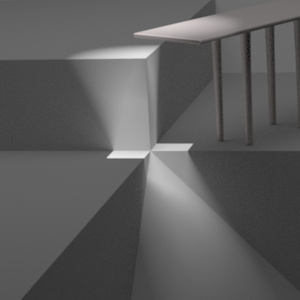
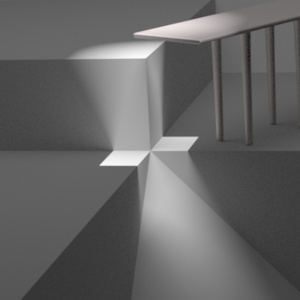
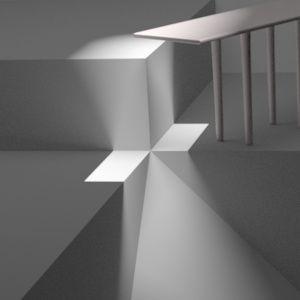
projector_type
Int enum
0 = “perspective” (default)
1 = “orthographic”
The projection type used to map points to the flap opening. Perspective has a focal point, while orthographic does not.
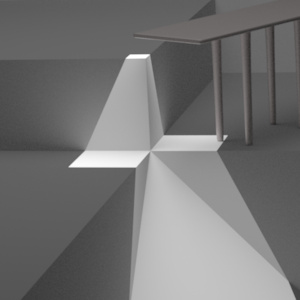
mode = analytical
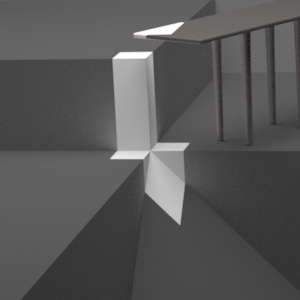
mode = analytical
projector_width
Float
default: 1.0
The width of the frustum at distance 1.0.
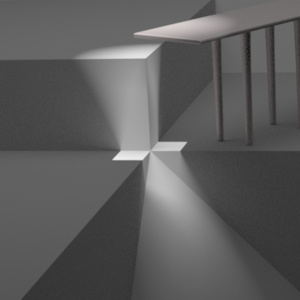
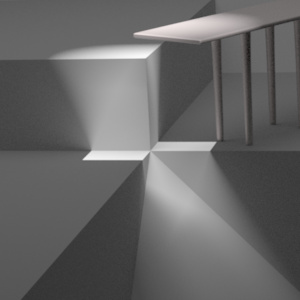
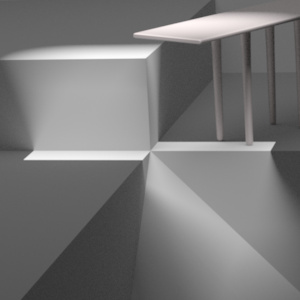
radius
Float
default: 0.0
The radius by which to convert the base box shape into a rounded box, as a proportion of half the width (or height, whichever is smaller).
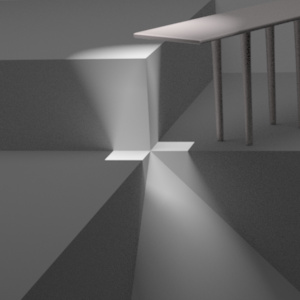
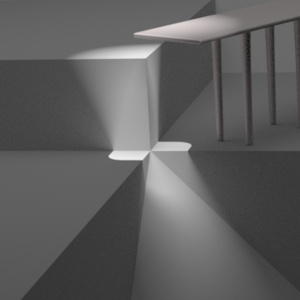
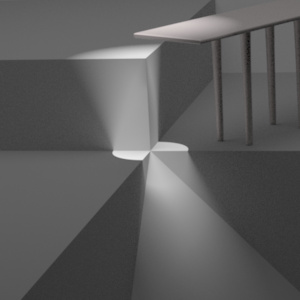
rotation
Float
default: 0.0
The angle to rotate the Barn Door counter-clockwise as seen from the light, in degrees.
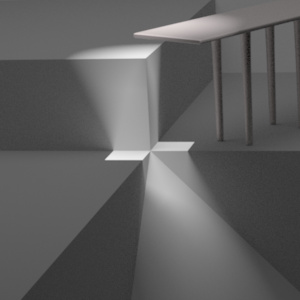
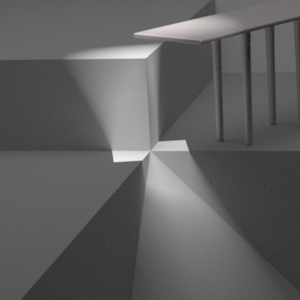
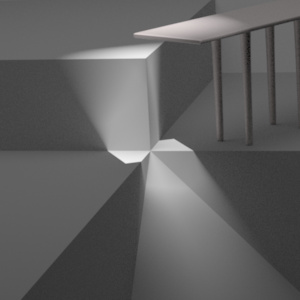
size_bottom
Float
default: 0.0
Additional size on the bottom edge.
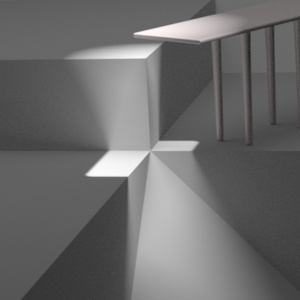
size_left
Float
default: 0.0
Additional size on the left edge.
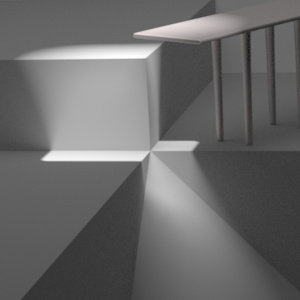
size_right
Float
default: 0.0
Additional size on the right edge.
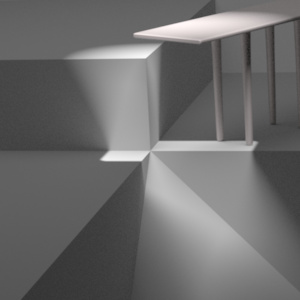
size_top
Float
default: 0.0
Additional size on the top edge.
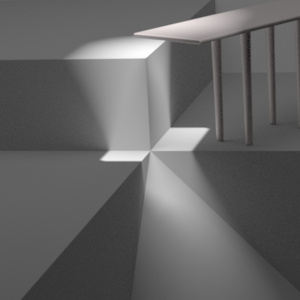
use_light_xform
Bool
default: True
Attach the filter to the light (in the -Z direction) and ignore node_xform.
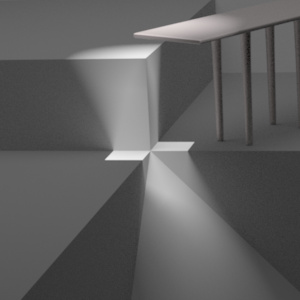
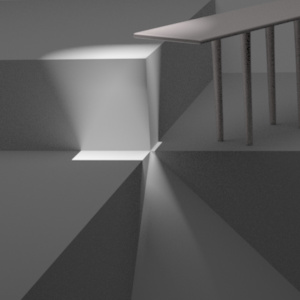
node_xform = rotate(-90,1,0,0) * translate(-2,9,-6)
General attributes
on
Bool
default: True
Turns the light filter on/off.
Examples
![]() |
![]() |
---|---|
No light filter | With BarnDoorLightFilter |
filter = BarnDoorLightFilter("/Scene/lighting/barndoor") {
["node_xform"] = Mat4(-0.0291956, 0, 0.999573, 0,
0.999573, 0, 0.0291956, 0,
0, 1, 0, 0,
0, 9, -7, 1),
["projector_width"] = 0.5,
["projector_height"] = 0.5,
["projector_type"] = "perspective",
["projector_focal_distance"] = 9,
["pre_barn_mode"] = "black",
["pre_barn_distance"] = 0,
["mode"] = "physical",
["invert"] = false,
["radius"] = 0.2,
["edge"] = 0.2,
["use_light_xform"] = true,
["edge_scale_top"] = 1.0,
["edge_scale_bottom"] = 0.11,
["edge_scale_left"] = 0.5,
["edge_scale_right"] = 10,
["rotation"] = 35,
["density"] = 0.95,
["color"] = Rgb(1,1,1),
["on"] = true,
["size_top"] = 0,
["size_bottom"] = 0,
["size_left"] = 0,
["size_right"] = 0,
}
In the following examples, a BarnDoorLightFilter is above the scene aiming straight down from a light. The focal length is such that the flap opening occurs exactly at the ground plane. The scene geometry is designed to illustrate the shape of the rectangular flap opening and the shape of the filtered light.
on
![]() |
![]() |
---|---|
on=true |
on=false |