NoiseWorleyMap_v2
Overview
NoiseWorleyMap creates procedural noise based on distance to randomly scattered points, creating a cellular effect.
Order of Operations:
When changing settings of this map, they’re applied in this order:
- Noise Calculation
- Bias
- Gain
- Smoothstep
- Amplitude
- Invert
Attribute Reference
Adjustment attributes
bias
Float bindable
default: 0.5
Bias of interpolation from color A to color B
gain
Float bindable
default: 0.5
Gain of interpolation from color A to color B
invert
Bool
default: False
Invert the final pattern
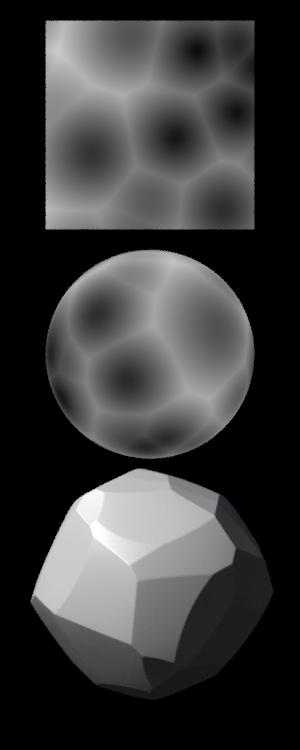
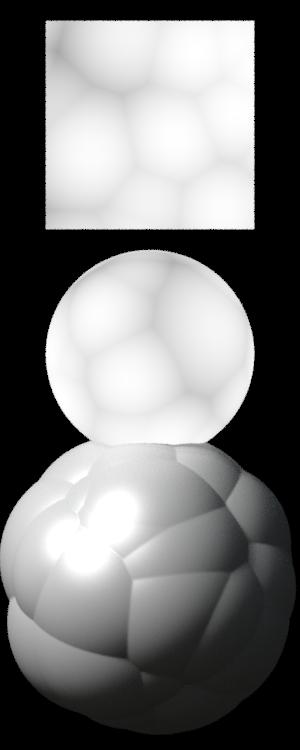
point_size
Float
default: 1.0
For points output mode, relative radius of points
remap
Vec2f bindable
default: [ 0, 1 ]
Allows mapping the distances from the specified min/max range into the 0..1 range
smoothstep
Vec2f bindable
default: [ 0, 1 ]
min/max values between which the smoothstep will interpolate
use_smoothstep
Bool
default: False
Put the noise value through a smoothstep function defined by min/max
Advanced attributes
F1
Float bindable
default: 1.0
Influence of F1 (the closest feature point)
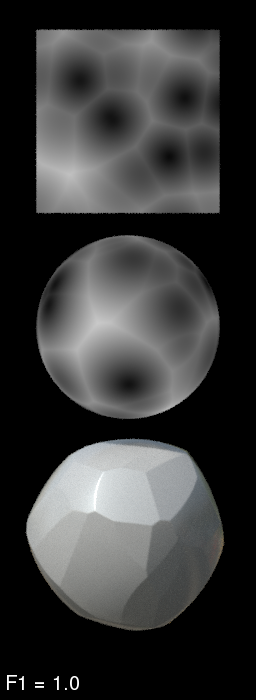
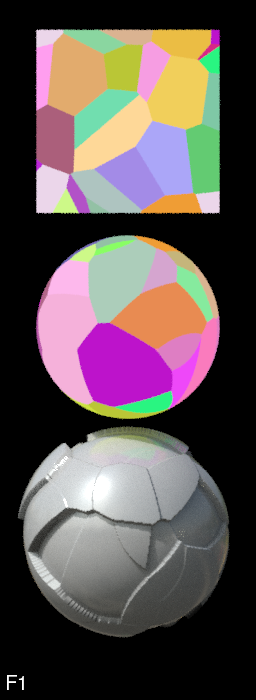
F2
Float bindable
default: 0.0
Influence of F2 (the second closest feature point)
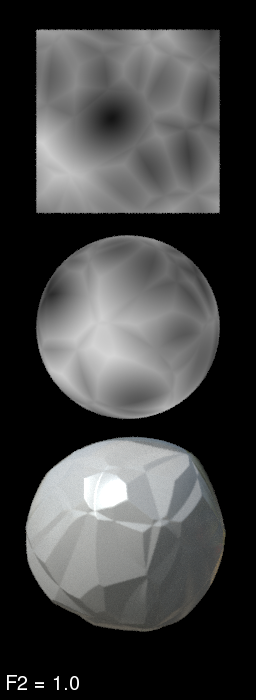
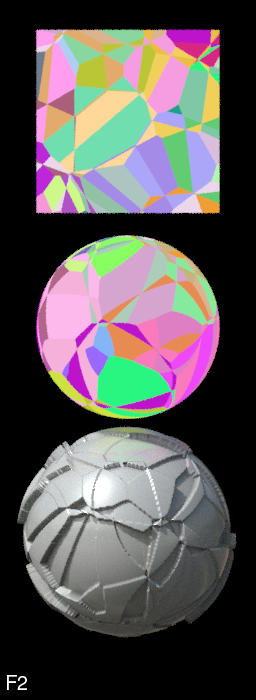
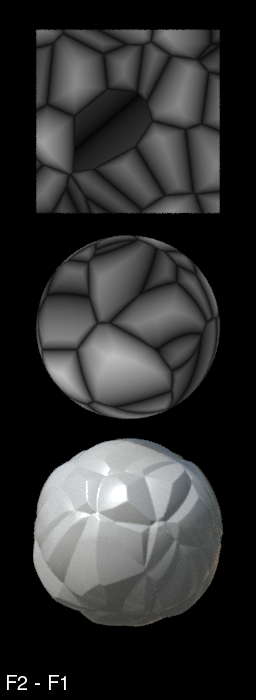
F3
Float bindable
default: 0.0
Influence of F3 (the third closest feature point)
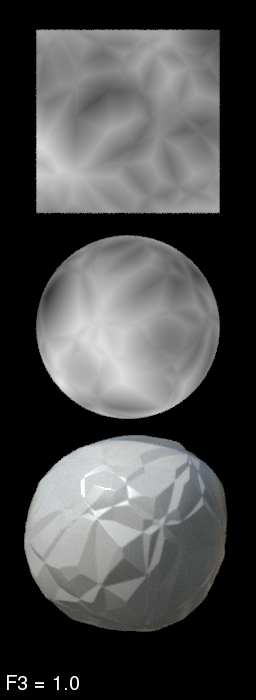
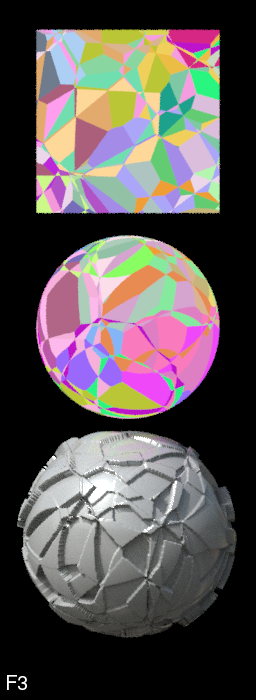
F4
Float bindable
default: 0.0
Influence of F4 (the fourth closest feature point)
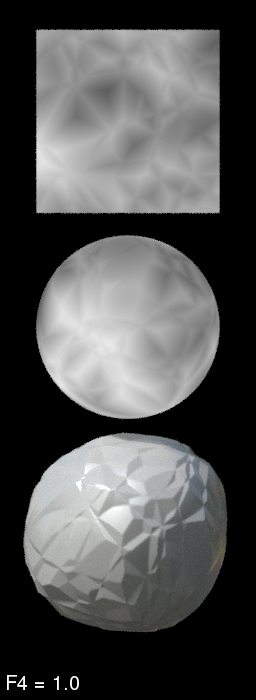
cell_id
Int enum
0 = “f1” (default)
1 = “f2”
2 = “f3”
3 = “f4”
Which of the distances determines the cell id
Output attributes
distance_method
Int enum
0 = “linear” (default)
1 = “linear squared”
2 = “manhattan”
3 = “chebyshev”
4 = “quadratic”
5 = “minkowski”
Metric for calculating distance to feature points which controls the shape of the falloff when output mode is distance
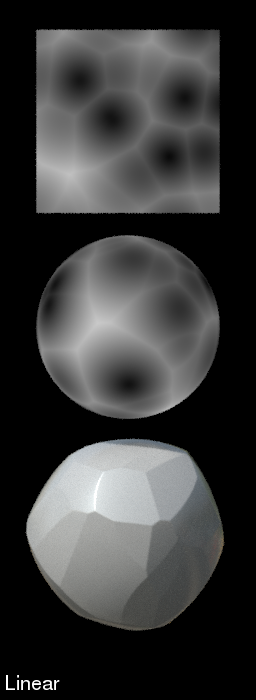
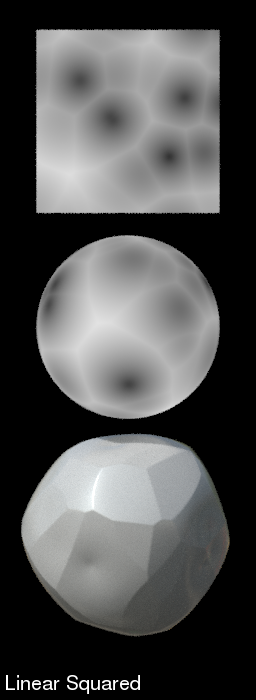
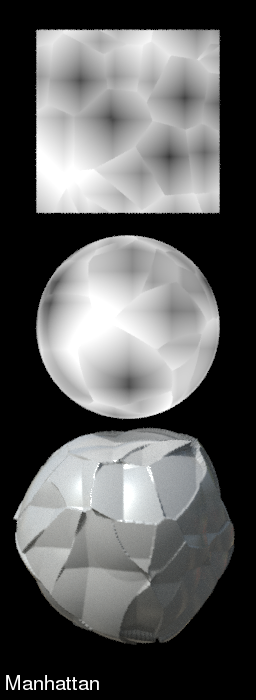
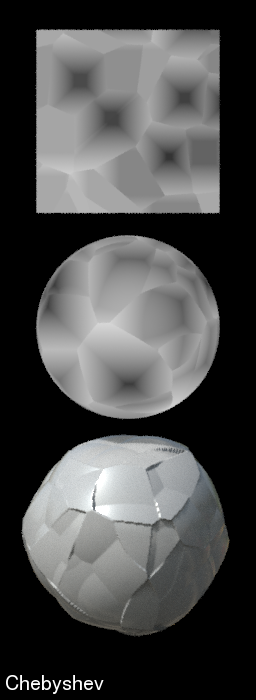
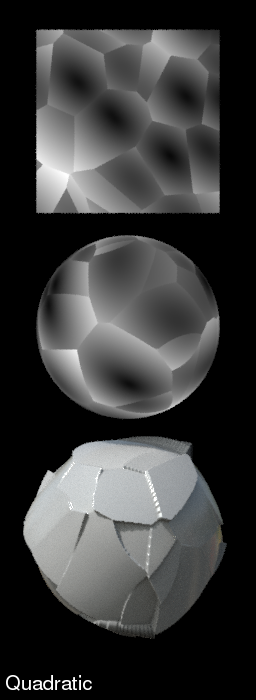
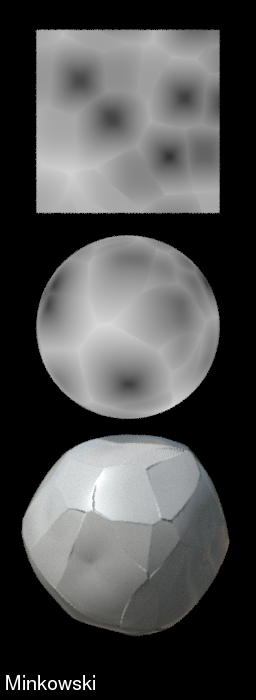
minkowski_number
Float bindable
default: 3.0
Exponent on distances when distance method is set to Minkowski
output_mode
Int enum
0 = “distance” (default)
1 = “gradient”
2 = “cell id”
3 = “cell edges”
4 = “points”
Method by which the shader outputs a color. Distance uses F1..F4 interpolated between color A and color B, gradient outputs the gradient of the noise, and cell ID outputs a random color for each cell
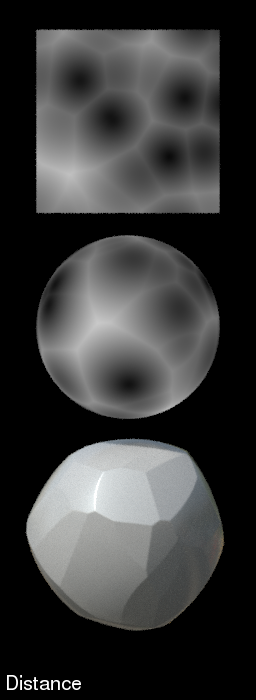
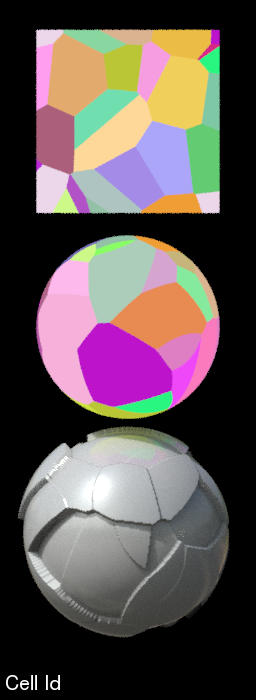
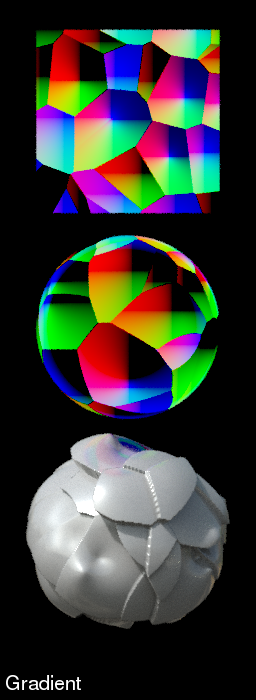
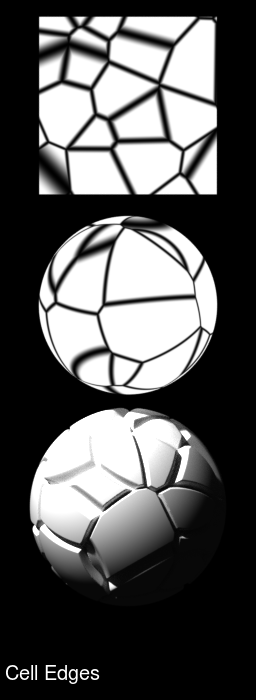
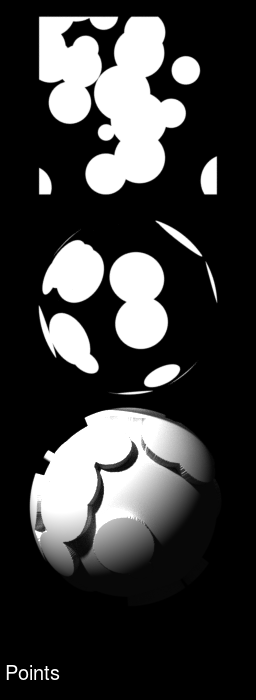
Space attributes
camera
Camera
default: None
Camera used to define camera and screen space
input_texture_coordinates
Vec3f bindable
default: [ 0, 0, 0 ]
User specified UVs
object_space
Geometry
default: None
Directly connect object to use that object's space.
space
Int enum
0 = “render”
1 = “camera”
2 = “world” (default)
3 = “screen”
4 = “object”
5 = “reference”
6 = “texture”
7 = “input texture coordinates”
8 = “hair_surface_uv”
9 = “hair_closest_surface_uv”
The space to calculate the noise in
Transform attributes
rotation
Vec3f bindable
default: [ 0, 0, 0 ]
Rotates the noise in space based on the specified rotation order
rotation_order
Int enum
0 = “xyz” (default)
1 = “xzy”
2 = “yxz”
3 = “yzx”
4 = “zxy”
5 = “zyx”
Order in which to apply the euler rotations
scale
Vec3f bindable
default: [ 1, 1, 1 ]
Vector to scale the noise non-proportionally
transformation_order
Int enum
0 = “srt”
1 = “str”
2 = “rst”
3 = “rts”
4 = “tsr” (default)
5 = “trs”
Order in which to apply the translation, rotation, and frequency
translation
Vec3f bindable
default: [ 0, 0, 0 ]
Translation of the noise in space
General attributes
color_A
Rgb bindable
default: [ 0, 0, 0 ]
The interpolated color value at distance equals zero
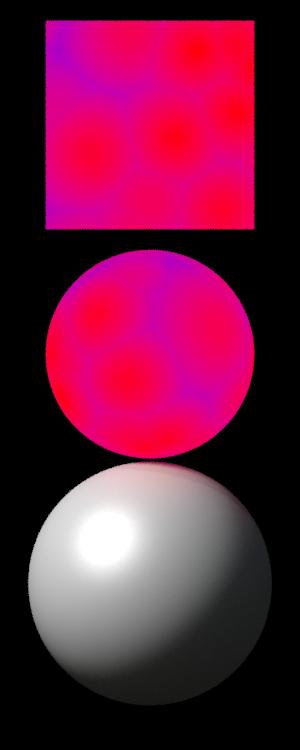
color_B
Rgb bindable
default: [ 1, 1, 1 ]
The interpolated color value at distance equals one
frequency
Float bindable
default: 1.0
Scalar multiplier for the frequency vector
jitter
Float bindable
default: 1.0
Controls the distortion of the cells
max_level
Float bindable
default: 1.0
Number of octaves of noise to add together for the final result
seed
Int
default: 0
The seed for the random number generator