ConvolutionDisplayFilter
Overview
The ConvolutionDisplayFilter convolves a kernel with a specified kernel_type
and kernel_size
with an input
image.
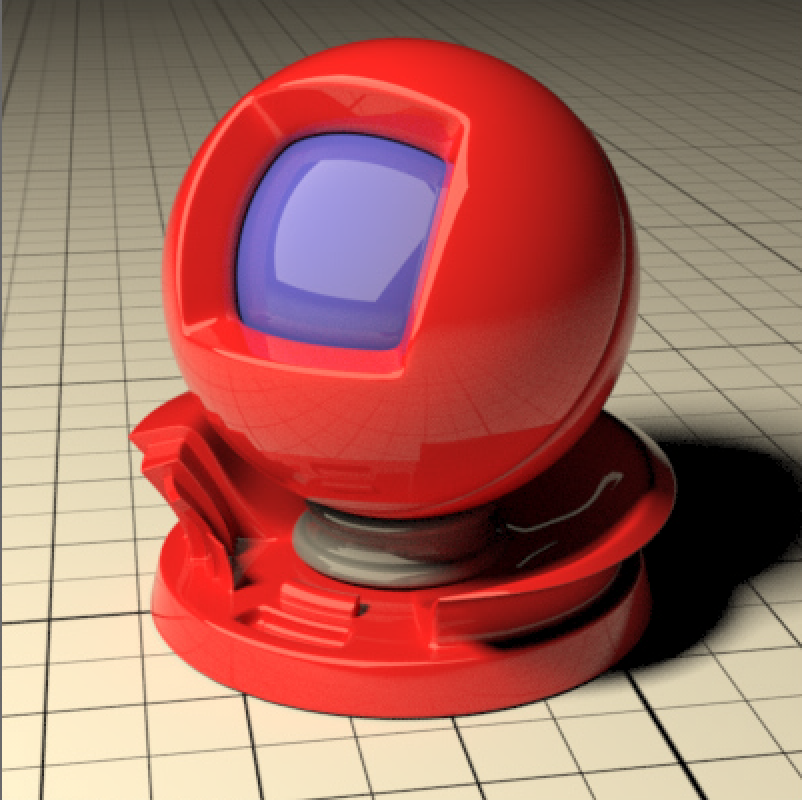
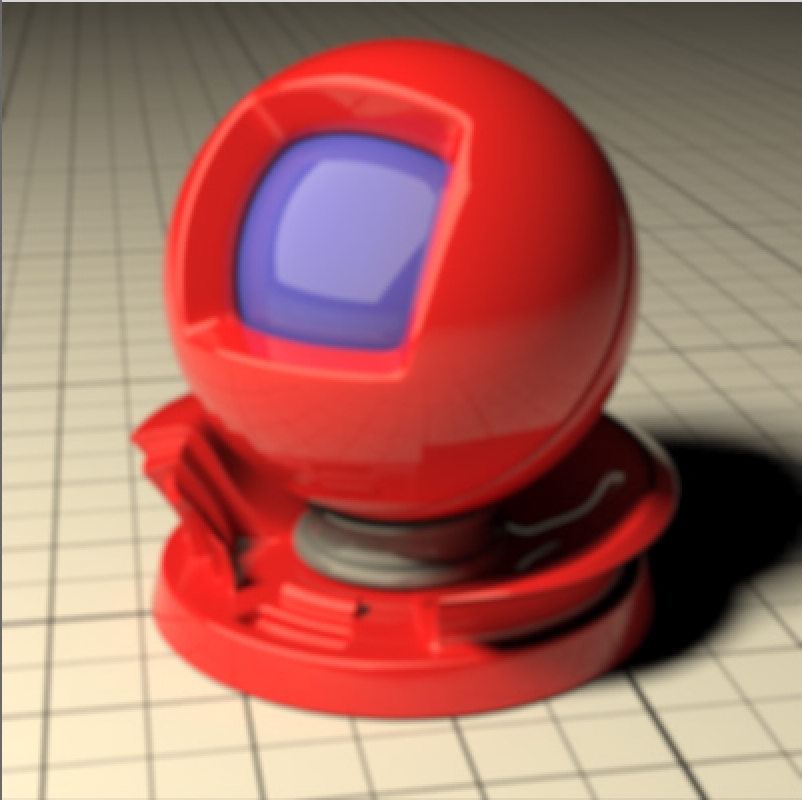
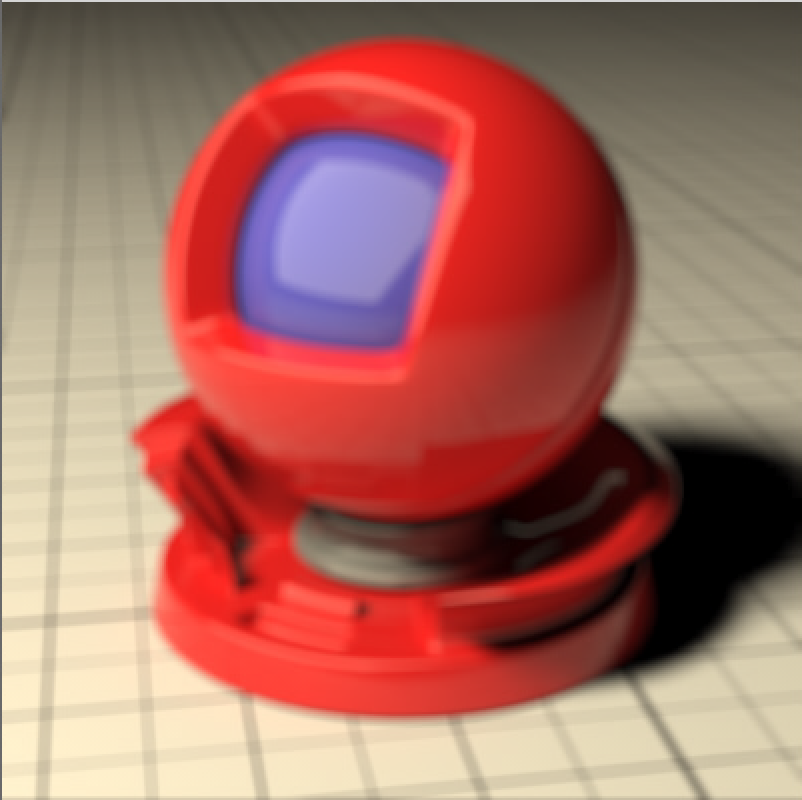
Attribute Reference
Advanced attributes
invert_mask
Bool
default: False
Invert the value of the mask
mix
Float
default: 1.0
Blend [0,1] between input and output
General attributes
custom_kernel
FloatVector
default: {}
A list of kernel values for a custom filter. The number of values provided must be the square of an odd number (e.g. 3x3, 5x5, 7x7).
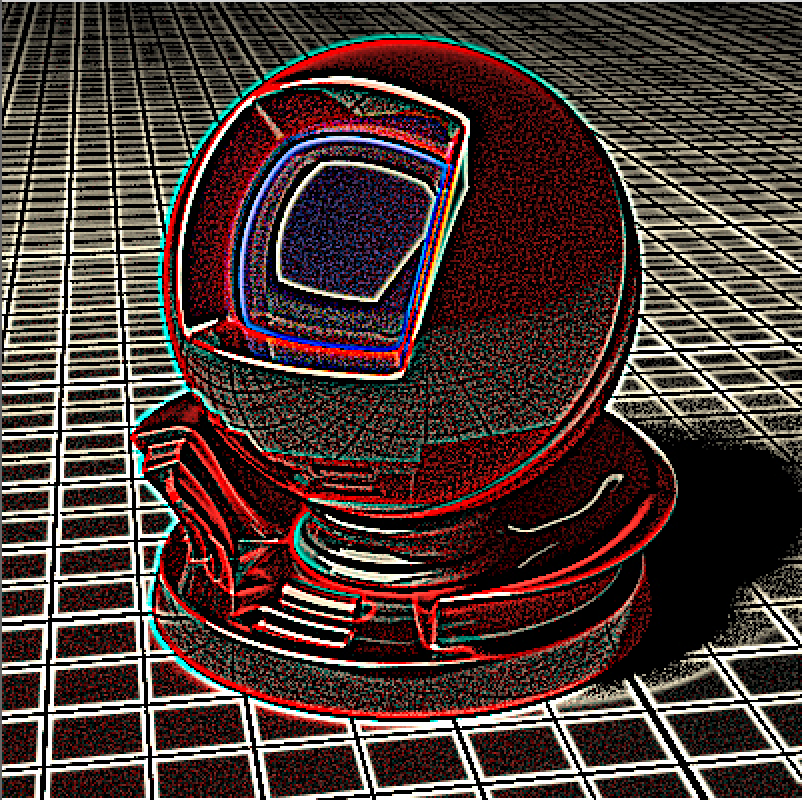
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, 24.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0}
input
RenderOutput
default: None
RenderOutput to convolve
kernel_size
Int
default: 5
Size of kernel in pixels. Size must be odd. If using a custom kernel, this attribute is ignored, and the size of the custom kernel is used instead.
kernel_type
Int enum
0 = “gaussian” (default)
1 = “box”
2 = “custom”
Kernel to use for convolution.
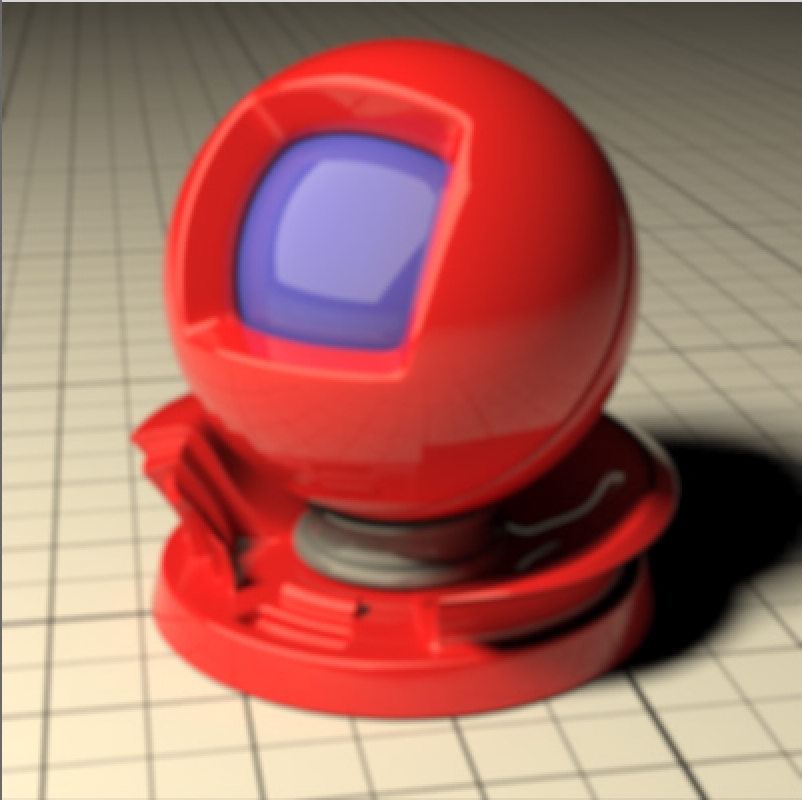
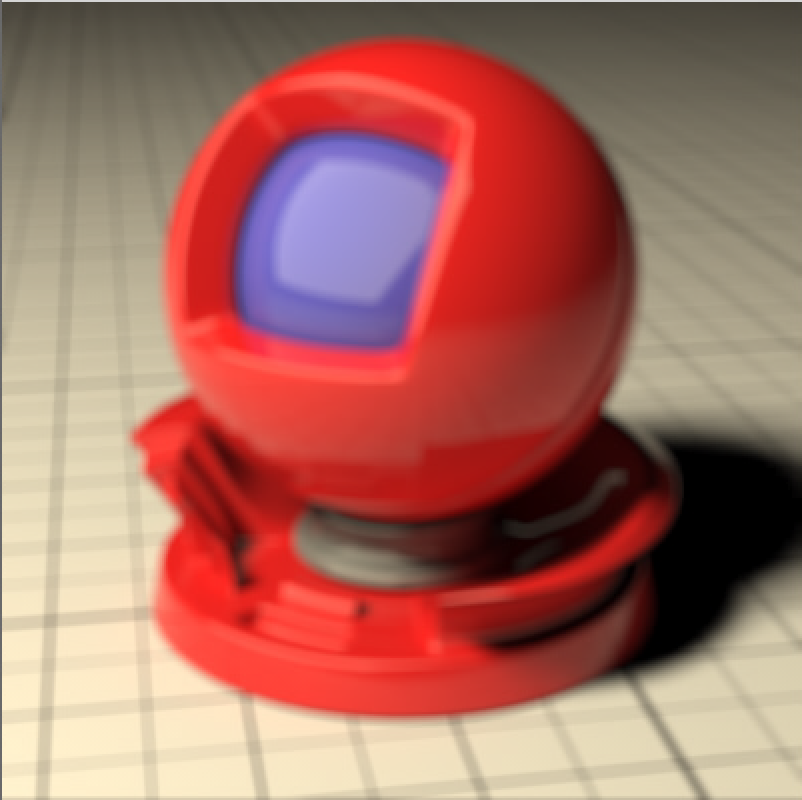
mask
RenderOutput
default: None
RenderOutput used to mask the output, revealing input1
Examples
local beauty = RenderOutput("/output/beauty") {
["file_name"] = "result_tmp.exr",
["result"] = "beauty",
}
local gaussian_filter = ConvolutionDisplayFilter("/display/gaussian") {
["input"] = beauty,
["kernel_type"] = "gaussian",
}
local laplacian_filter = ConvolutionDisplayFilter("/display/laplacian") {
["input"] = beauty,
["kernel_type"] = "custom",
["custom_kernel"] = {
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, 24.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0,
-1.0, -1.0, -1.0, -1.0, -1.0}
}
RenderOutput("/output/guassian") {
["file_name"] = "result0.exr",
["result"] = "display filter",
["display_filter"] = gaussian_filter,
["channel_name"] = "gaussian"
}
RenderOutput("/output/laplacian") {
["file_name"] = "result0.exr",
["result"] = "display filter",
["display_filter"] = laplacian_filter,
["channel_name"] = "laplacian"
}