ProjectTriplanarNormalMap
Overview
ProjectTriplanarNormalMap uses the normals and position on a mesh to apply and blend normal textures procedurally.
The triplanar projection is a virtual cube, where textures repeat along each cube face. The blending weight for each face’s texture is determined by the face’s alignment with the mesh normal. The transition_width
parameter increases or decreases the amount of blending across faces. The normal map is applied with the correct tangent for each face for the cube.
The number_of_textures
option also determines how the projection works:
one
texture means it’s the same on all facesthree
textures means the X, Y, Z planes can each have their own texturesix
textures means positive and negative X, Y, Z can have unique textures
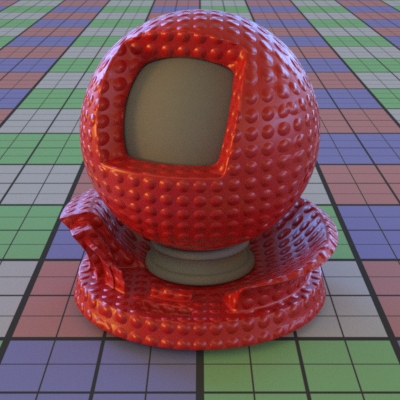
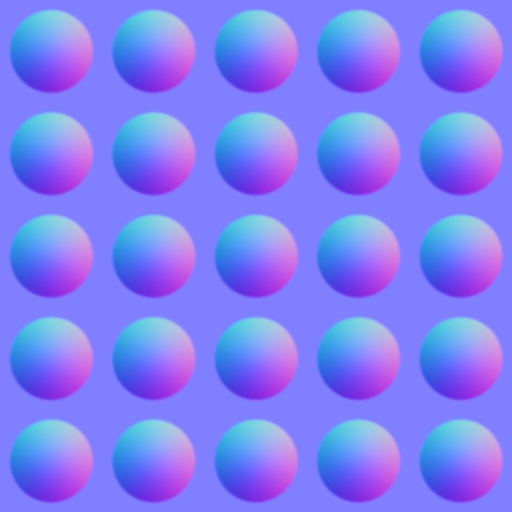
Attribute Reference
Negative X Modifiers attributes
negative_x_invert_s
Bool
default: False
Flip in the s direction (horizontal)
negative_x_invert_t
Bool
default: False
Flip in the t direction (vertical)
negative_x_offset
Vec2f
default: [ 0, 0 ]
2D offset
negative_x_rotation
Float
default: 0.0
2D rotation amount
negative_x_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
negative_x_scale
Vec2f
default: [ 1, 1 ]
2D scale
negative_x_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
negative_x_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
Negative Y Modifiers attributes
negative_y_invert_s
Bool
default: False
Flip in the s direction (horizontal)
negative_y_invert_t
Bool
default: False
Flip in the t direction (vertical)
negative_y_offset
Vec2f
default: [ 0, 0 ]
2D offset
negative_y_rotation
Float
default: 0.0
2D rotation amount
negative_y_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
negative_y_scale
Vec2f
default: [ 1, 1 ]
2D scale
negative_y_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
negative_y_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
Negative Z Modifiers attributes
negative_z_invert_s
Bool
default: False
Flip in the s direction (horizontal)
negative_z_invert_t
Bool
default: False
Flip in the t direction (vertical)
negative_z_offset
Vec2f
default: [ 0, 0 ]
2D offset
negative_z_rotation
Float
default: 0.0
2D rotation amount
negative_z_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
negative_z_scale
Vec2f
default: [ 1, 1 ]
2D scale
negative_z_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
negative_z_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
Positive X Modifiers attributes
positive_x_invert_s
Bool
default: False
Flip in the s direction (horizontal)
positive_x_invert_t
Bool
default: False
Flip in the t direction (vertical)
positive_x_offset
Vec2f
default: [ 0, 0 ]
2D offset
positive_x_rotation
Float
default: 0.0
2D rotation amount
positive_x_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
positive_x_scale
Vec2f
default: [ 1, 1 ]
2D scale
positive_x_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
positive_x_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
Positive Y Modifiers attributes
positive_y_invert_s
Bool
default: False
Flip in the s direction (horizontal)
positive_y_invert_t
Bool
default: False
Flip in the t direction (vertical)
positive_y_offset
Vec2f
default: [ 0, 0 ]
2D offset
positive_y_rotation
Float
default: 0.0
2D rotation amount
positive_y_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
positive_y_scale
Vec2f
default: [ 1, 1 ]
2D scale
positive_y_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
positive_y_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
Positive Z Modifiers attributes
positive_z_invert_s
Bool
default: False
Flip in the s direction (horizontal)
positive_z_invert_t
Bool
default: False
Flip in the t direction (vertical)
positive_z_offset
Vec2f
default: [ 0, 0 ]
2D offset
positive_z_rotation
Float
default: 0.0
2D rotation amount
positive_z_rotation_center
Vec2f
default: [ 0, 0 ]
2D rotation center
positive_z_scale
Vec2f
default: [ 1, 1 ]
2D scale
positive_z_swap_st
Bool
default: False
Swap the s and t directions. Same as a 90 degree rotation
positive_z_wrap_around
Bool
default: True
Controls whether to repeat (true) or clamp (false) the texture
General attributes
TRS_order
Int enum
0 = “Scale Rot Trans” (default)
1 = “Scale Trans Rot”
2 = “Rot Scale Trans”
3 = “Rot Trans Scale”
4 = “Trans Scale Rot”
5 = “Trans Rot Scale”
Order in which to apply transformations when 'projection_mode' is set to 'TRS'
negative_x_active
Bool
default: True
Turns this direction on/off. Output is black if off.
negative_x_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
negative_y_active
Bool
default: True
Turns this direction on/off. Output is black if off.
negative_y_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
negative_z_active
Bool
default: True
Turns this direction on/off. Output is black if off.
negative_z_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
normal_encoding
Int enum
0 = “[0,1]” (default)
1 = “[-1,1]”
Most normal maps are encoded [0,1]. Only certain rare floating point normal maps are encoded [-1,1]
number_of_textures
Int enum
1 = “one”
3 = “three” (default)
6 = “six”
Controls the number of active textures. If set to 'one', only the 'pos x' texture settings will be used for all sides. If set to 'three' the pos x, pos y, and pos z settings will be used for their respective negative sides. If set to 'six', each side has independent controls and texture.
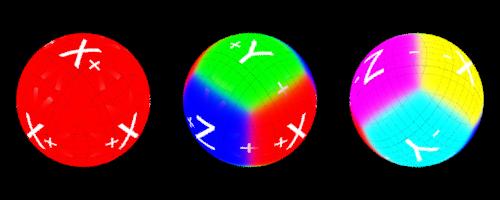
positive_x_active
Bool
default: True
Turns this direction on/off. Output is black if off.
positive_x_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
positive_y_active
Bool
default: True
Turns this direction on/off. Output is black if off.
positive_y_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
positive_z_active
Bool
default: True
Turns this direction on/off. Output is black if off.
positive_z_texture
String filename
default:
Filename that points to a texture .exr or .tx file (must be mip-mapped and tiled with maketx).
projection_matrix
Mat4d
default: [ [ 1, 0, 0, 0 ], [ 0, 1, 0, 0 ], [ 0, 0, 1, 0 ], [ 0, 0, 0, 1 ] ]
The transform to use for projection when 'projection_mode' is set to 'projection_matrix'
projection_mode
Int enum
0 = “projector” (default)
1 = “projection_matrix”
2 = “TRS”
Source parameters to use for projection transform
projector
Node
default: None
The object whose transform to use for projection when 'projection_mode' is set to 'projector'
random_seed
Int
default: 8241
Seed for randomizing orientation, offset, and flip
randomize_flip
Bool
default: False
Random flipping in S or T for each active texture

randomize_offset
Bool
default: False
Random offset in S or T for each active texture

randomize_rotation
Bool
default: False
Random 2d rotation of each active texture

rotate
Vec3d
default: [ 0, 0, 0 ]
Rotation of the projection transform when 'projection_mode' is set to 'TRS'
rotation_order
Int enum
0 = “xyz” (default)
1 = “xzy”
2 = “yxz”
3 = “yzx”
4 = “zxy”
5 = “zyx”
Order in which to apply rotation transformations when 'projection_mode' is set to 'TRS'
scale
Vec3d
default: [ 1, 1, 1 ]
Scale of the projection transform when 'projection_mode' is set to 'TRS'
transition_width
Float
default: 0.5
Controls blending of per-axis projections. Valid range is 0.0 (no blending) to 1.0 (max blending)
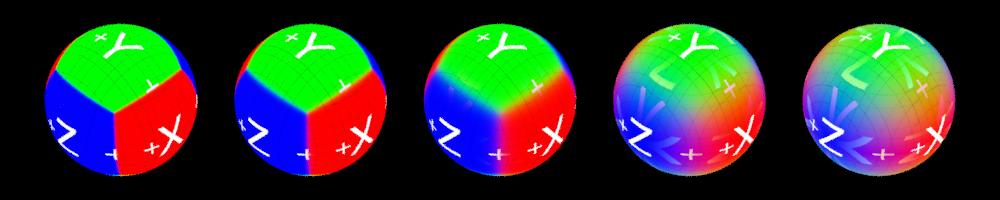
translate
Vec3d
default: [ 0, 0, 0 ]
Translation of the projection transform when 'projection_mode' is set to 'TRS'
use_reference_space
Bool
default: False
Project onto reference positions ('ref_P') and normals ('ref_N')